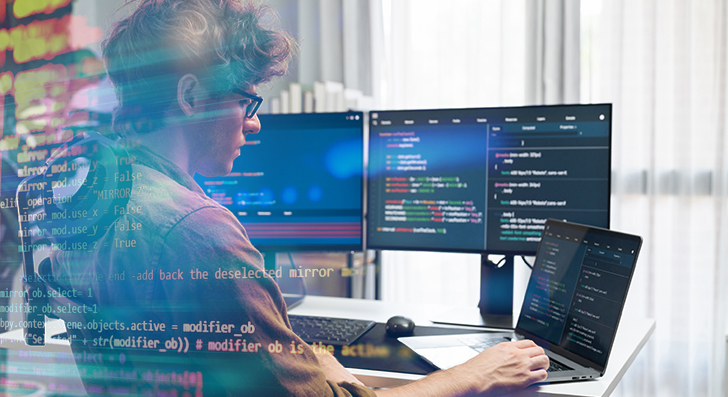
Scalability indicates your application can deal with growth—extra people, far more information, and much more website traffic—with no breaking. As being a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be component within your program from the start. Several purposes fall short every time they expand fast due to the fact the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your system will behave under pressure.
Begin by coming up with your architecture to become versatile. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular design or microservices. These patterns crack your app into more compact, unbiased parts. Each and every module or assistance can scale on its own with out impacting the whole program.
Also, contemplate your databases from day a single. Will it need to have to take care of one million customers or perhaps a hundred? Select the ideal type—relational or NoSQL—determined by how your details will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them yet.
A different vital stage is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present situations. Think of what would materialize When your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure patterns that support scaling, like message queues or event-pushed programs. These support your app deal with much more requests without having acquiring overloaded.
If you Construct with scalability in mind, you're not just getting ready for success—you are reducing long term headaches. A well-prepared process is easier to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the proper Databases
Deciding on the appropriate database is often a essential Portion of developing scalable applications. Not all databases are built the same, and utilizing the Erroneous one can slow you down or simply cause failures as your application grows.
Start off by knowing your data. Can it be very structured, like rows inside a desk? If Of course, a relational database like PostgreSQL or MySQL is a good match. These are potent with associations, transactions, and consistency. Additionally they assistance scaling procedures like read through replicas, indexing, and partitioning to handle additional website traffic and info.
In the event your knowledge is more versatile—like user action logs, product catalogs, or paperwork—consider a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and may scale horizontally extra effortlessly.
Also, take into consideration your go through and generate patterns. Will you be undertaking many reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Consider databases that will cope with high compose throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not want Highly developed scaling attributes now, but selecting a database that supports them implies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data dependant upon your entry styles. And generally observe databases general performance when you mature.
To put it briefly, the right databases relies on your app’s structure, speed needs, And exactly how you hope it to mature. Take time to select correctly—it’ll help save a great deal of difficulties later on.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, every compact hold off adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s imperative that you Make successful logic from the start.
Start by crafting cleanse, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most sophisticated solution if a straightforward one particular functions. Keep the features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places where your code can take also long to operate or utilizes far too much memory.
Up coming, look at your databases queries. These often sluggish things down in excess of the code by itself. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from performing a lot of joins, especially across substantial tables.
In the event you detect the same knowledge remaining requested over and over, use caching. Retail store the results quickly using equipment like Redis or Memcached this means you don’t need to repeat pricey functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app additional efficient.
Remember to check with massive datasets. Code and queries that get the job done fine with 100 records may well crash whenever they have to take care of one million.
To put it briefly, scalable applications are quickly apps. here Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more end users and a lot more website traffic. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment support maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors across multiple servers. Instead of a person server accomplishing the many get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If just one server goes down, the load balancer can ship traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When end users request a similar information yet again—like a product web site or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) outlets info in memory for speedy entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching decreases databases load, improves pace, and makes your application more successful.
Use caching for things that don’t transform often. And constantly make sure your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of extra buyers, stay rapidly, and Get better from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your app increase quickly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may center on building your application in lieu of running infrastructure.
Containers are A different critical Device. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, from the laptop computer for the cloud, with out surprises. Docker is the most popular Resource for this.
Whenever your app takes advantage of a number of containers, resources like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different aspects of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to increase without boundaries, get started making use of these applications early. They conserve time, lower risk, and allow you to continue to be focused on constructing, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when issues go Mistaken. Checking aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a essential Component of building scalable methods.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this details.
Don’t just observe your servers—monitor your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant complications. Such as, In the event your reaction time goes above a Restrict or simply a company goes down, you'll want to get notified straight away. This can help you deal with troubles rapidly, usually just before customers even notice.
Checking is likewise valuable once you make modifications. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it triggers actual harm.
As your application grows, targeted traffic and info increase. Devoid of monitoring, you’ll miss indications of difficulty right until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about comprehension your method and making certain it works well, even under pressure.
Final Ideas
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By coming up with cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to Establish apps that increase smoothly without having breaking stressed. Begin modest, Imagine huge, and Make smart.